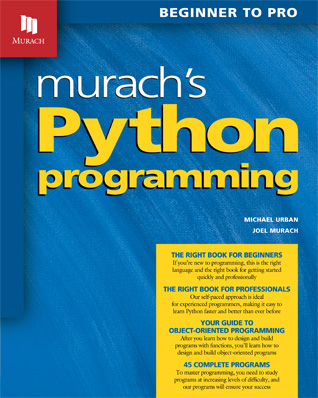
Because of its simple syntax, full set of features, and wide range of applications, Python is an ideal language for a first programming course. And our book takes advantage of the Python syntax and features to help beginners learn how to program faster and better than ever. Along the way, they’ll master the skills that are common to all programming languages so that learning the next language, like Java or C#, will be much easier. And at the end, they’ll have the basic Python skills that are in such demand today for applications ranging from web and game development to data analysis, scientific computing, artificial intelligence…and more!
Python Programming (2nd Edition)
Published April 2021
This is by far the best Python tutorial I have come across. Tried Udemy, Udacity, some other video and printed tutorials, but didn’t get the feel of it. Murach's side-by-side lecture & example really works for me. And the sample codes and exercises are valuable tutorials that any newbie will want to keep as reference materials. Really effective training style.”
To present the Python skills in a manageable progression and at the right pace, this book is divided into four sections.
We’re familiar with the competing Python books, and we’ve specifically designed this book to help your students learn faster and better than they can with any of them. Here are a few of the ways it does that:
“I've been a fan of Murach's approach to teaching programming concepts and practices for many years. Now they've finally published a book on Python, and they've done a wonderful job. The book explores a wide breadth of topics to get you competent in the language and the conventions used by the Python community. It largely sticks to examples of building Python programs for the console, which shows how easy it is to get up and running. A valuable resource for coders of all levels.”
- Jason Salas, Developer
“Best programming book on Python. You'll learn to love Murach's books. I learned Python and now I'm learning Java. I bought Murach's Java book even though we're using another one for class.”
- Posted online by student
“I just received your Python programming book which is just great - like all of your books are, to be honest. I'm glad it finally found its place in your library.”
- Luka Kolonic, IT Professional, Slovenia
“I found this to be a fantastic book for taking folk with no Python knowledge to a good firm grasp to develop their own projects, as well as folk with some knowledge who want to shore up the gaps.
“Each chapter does a really great job succinctly explaining the concept, why you would use it, has relevant code, and great summary/exercises to reinforce the area. The code is clean, well formatted, and uses great naming conventions. The projects and examples are spot on.
“As a moderate Python user, I definitely learned a few new tricks, and reduced some complexity in my code. I have no qualms recommending this book for folk looking to pick up Python and use it!”
- Jeremy Johnson, Dream in Code
“Murach isn't a publisher I was familiar with so I was surprised when I started reading its Python Programming book and found it to be of such high quality. I feel like it covered the topics well and included some items that you don't always see in introductory Python books, such as the CSV, database and GUI examples…. The text is clear and the examples are interesting. I really like that you get to write mini-programs in almost all of the chapters and they almost always do something besides just printing out a string.
“I believe this is a worthy book for someone who wants to learn Python.”
- Mike Driscoll, Programmer, Mouse vs Python blog
View the table of contents for this book in a PDF: Table of Contents (PDF)
Click on any chapter title to display or hide its content.
Why Python works so well as your first programming language
Three types of Python applications
The source code for a console application
How Python compiles and runs source code
How disk storage and main memory work together
How to use the interactive shell
How to work with source files
How to compile and run a program
How to fix syntax and runtime errors
How to code statements
How to code comments
How to use functions
How to assign values to variables
How to name variables
How to code arithmetic expressions
How to use arithmetic expressions in assignment statements
How to use the interactive shell for testing numeric operations
How to assign strings to variables
How to join strings
How to include special characters in strings
How to use the interactive shell for testing string operations
How to use the print() function
How to use the input() function
How to use the int(), float(), and round() functions
How to chain functions
The Miles Per Gallon program
The Test Scores program
How to use the relational operators
How to use the logical operators
How to compare strings
How to code if statements
More examples of if statements
How to code nested if statements
How to use pseudocode to plan if statements
The Miles Per Gallon program
The Invoice program
How to code while statements
How to code for statements
How to code break and continue statements
More examples of loops
How to use pseudocode to plan a program
The Test Scores program
The Future Value program
How to define and call a function
How to define and call a main() function
The Future Value program with functions
How to use default values for arguments
How to use named arguments
When and how to use local and global variables
How to create a module
How to document a module
How to import a module
The Convert Temperatures program
How to use the random module
The Guess the Number game
How to use a hierarchy chart
The hierarchy chart for the Pig Dice game
The Pig Dice game with global variables
The Pig Dice game with local variables
The three types of errors that can occur
Common Python errors
How to plan the test runs
A simple way to trace code execution
How to use top-down coding and testing to simplify debugging
How to use the IDLE shell to test functions
How to set and remove breakpoints
How to step through the code
How to view the stack
How to create a list
How to get and set items
How to add and remove items
How to process the items in a list
How lists are passed to functions
The Movie List program
How to create a list of lists
How to process the items in a list of lists
The Movie List 2D program
How to count, reverse, and sort the items in a list
How to use other functions with lists
How to copy, slice, and concatenate lists
How to create a tuple
How to get items from a tuple
The Number Crunching program
How file I/O works
How to open and close a file
How to write a text file
How to read a text file
How to work with a list in a text file
The Movie List 1.0 program
How to write a CSV file
How to read a CSV file
How to modify the CSV format
The Movie List 2.0 program
How to work with a binary file
The Movie List 3.0 program
How exceptions work
How to use a try statement to handle one type of exception
The Total Calculator program
How to use a try statement to handle multiple exceptions
How to get the information from an exception object
The Movie List 2.0 program
How to use a finally clause
How to raise an exception
How floating-point numbers work
How to use the math module
How to use the format() method of a string
How to use the locale module
How to fix rounding errors
How to use the decimal module
The Invoice program with decimal numbers
The Future Value program with decimal numbers
Unicode, indexes, slicing, duplicating, and multiline strings
How to search a string
How to loop through the characters in a string
How to use basic string methods
How to find and replace parts of a string
The Create Account program
How to split a string into a list of strings
How to join strings
The Movie List 2D program
The Word Counter program
The user interface
The hierarchy chart
The wordlist module
The hangman module
How to create date, time, and datetime objects
How to create datetime objects by parsing strings
How to format dates and times
How to work with spans of time
The Invoice Due Date program
The Timer program
How to get date and time parts
How to compare date/time objects
The Hotel Reservation program
How to create a dictionary
How to get, set, and add items
How to delete items
How to loop through keys and values
How to convert between dictionaries and lists
The Country Code program
The Word Counter program
How to use dictionaries with complex objects as values
The Book Catalog program
How recursion works in Python
How to use recursion to add a range of numbers
How to compute the factorial of a number
How to compute a Fibonacci series
An algorithm for solving the Towers of Hanoi puzzle
The code for solving the Towers of Hanoi puzzle
Two UML diagrams for the Product class
Code that defines a Product class
Code that uses a Product class
How to create and use objects
How to code a constructor and attributes
How to code methods
The Product Viewer 1.0 program
How object composition works
The Die and Dice classes
The Dice Roller 1.0 program
How object encapsulation works
How to hide attributes
How to access hidden attributes with methods
How to access hidden attributes with properties
The Die and Dice classes with encapsulation
The Product class with some encapsulation
The console
The code
How inheritance works
How to define a subclass
How polymorphism works
How to check an object’s type
The objects module
The user interface and product_viewer module
How to define a string representation for an object
How to define an iterator for an object
The Die and Dice classes
How to work with custom exceptions
When to use inheritance
Five steps for designing an object-oriented program
How to identify the data attributes
How to subdivide the data attributes
How to identify the classes
How to identify the methods
How the three-tier architecture works
The business tier
The database tier
The presentation tier
How a database table is organized
How the tables in a database are related
How the columns in a table are defined
How to select data from a single table
How to select data from multiple tables
How to insert, update, and delete rows
How to connect to a SQLite database
How to execute SQL statements
How to connect to a SQLite database
How to execute SELECT statements
How to get the rows in a result set
How to execute INSERT, UPDATE, and DELETE statements
How to test the database code
How to handle database exceptions
The user interface
The business tier
The database tier
The presentation tier
How to display a root window
How to work with frames and buttons
How to handle a button click event
How to work with labels and text entry fields
How to lay out components in a grid
How to code a class that defines a frame
The business module
The ui module
How to install the source code for this book
How to install Python and IDLE
How to install Firefox and SQLite Manager
How to verify that the database is installed correctly
How to install the source code for this book
How to install Python and IDLE
How to verify that Python and IDLE are working correctly
How to install Firefox and SQLite Manager
How to verify that the database is installed correctly
In contrast to other college publishers, we don’t fill dozens of pages in our books with end-of-chapter (EOC) activities that may never be used. Instead, we provide everything you need for an effective course in a download from our instructor’s website. Then, you decide which of these materials you want to use.
What follows is a brief summary of the instructor’s materials for this book; for a detailed description in PDF, please read the Instructor's Summary. As we see it, these materials provide everything that you need for running a great course...without the counter-productive busywork of other texts.
To view the "Frequently Asked Questions" for this book in a PDF, just click on this link: View the questions
Then, if you have any questions that aren't answered here, please email us. Thanks!
To view the corrections for this book in a PDF, just click on this link: View the corrections
Then, if you find any other errors, please email us so we can correct them in the next printing of the book. Thank you!
This is our site for college instructors. To buy Murach books, please visit our retail site.