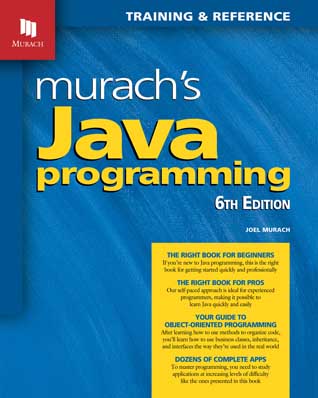
Now covers Java SE 17
Ever since the first edition of this book was published in 2001, it’s been a favorite of instructors and students because of the clear, concise way it teaches Java and object-oriented programming. Now, this 6th Edition has been updated, reorganized, and streamlined to focus on the essentials and to do a better job than ever of setting the right pace for a beginning Java student.
The Canvas course file contains all the objectives, quizzes, assignments, and slides that you need to run an effective course. It only takes a few clicks to import it into the Canvas LMS. Then, you can customize it for your course. Learn more.
I love the way this book is set up. We used this in my Java Programming class, and it just makes everything so much easier and clearer.”
This book focuses on what a beginning Java programmer needs to know. The Table of Contents shows how the content is structured. But briefly, here‘s how the content works for your students:
By the end of chapter 2, your students will be writing their first complete Java applications that get input, perform calculations, and display output.
By the end of chapter 9, your students will be writing object-oriented applications that validate user input and use files to store their data.
Starting from chapter 1, your students will take advantage of the time-saving features that an IDE provides as they use NetBeans or Eclipse to create, compile, run, test, and debug Java applications.
Chapter 7 introduces your students to object-oriented programming (OOP), including the concept of encapsulation. Then, chapters 10 and 11 explain inheritance and polymorphism in a practical way that cuts through the mystery and confusion these subjects can cause. This provides the foundation that your students need to develop real-world, object-oriented, business applications.
Section 3 shows how to develop attractive graphical user interfaces (GUIs) with JavaFX, a modern library that’s designed to replace the aging Swing library.
Section 4 presents more Java skills that your students will use all the time, like extra skills for working with strings, collections, lambdas, recursion, algorithms, dates, times, and databases. Each chapter in this section is independent of the others, so you can teach these chapters in whatever sequence you prefer.
Chapter 19 shows your students how to write database classes that map objects to a relational database. First, they’ll learn how to use SQL to work with a SQLite database. Then, they’ll learn how to use JDBC to work with any database.
By the end of section 2, your students will have the foundation in Java programming that they need to learn how to develop applications for the web or mobile devices. So, they’ll be ready for other courses that show how to use Java to develop web applications or Android apps.
We’ve specifically designed this book to help your students learn Java faster and better than they ever could before. Here are a few of the ways it does that:
Although this book covers Java SE 17, the code it presents will work with future versions of Java. In addition, this book clearly notes which features have been added since SE 9, which makes it possible to use this book with earlier versions too.
Using the time-saving features of an IDE is the easiest and best way to learn Java. That’s why this book shows how to use NetBeans and Eclipse, the two most popular IDEs for Java development.
Appendix A (Windows) and Appendix B (macOS) explain how to install the software, all of which is free.
This book has been updated from Java SE 9 to Java SE 17, which is the current long-term support (LTS) release. As part of this update, we have streamlined this book to do a better job than ever of setting the right pace for a beginning Java student.
“I love this book. It was the required text for my Java class and I'm glad. It's very concise, very easy to follow. The left page will be a detailed description of the figure on the page to the right, along with a breakdown of major takeaways from that section.”
- Posted at an online bookseller
“I initially rented this book for a crash course on Java GUI. I liked it so much that I ended up buying a copy.”
- Posted at an online bookseller
“One of the things I really like is that this book uses an IDE to teach Java to the next generation of programmers. A lot of books focus on the language itself [as though] an IDE plays no role in learning Java. Any professional programmer will tell you that an IDE is absolutely essential in making you more productive.”
- John Yeary, Java Evangelist
“Another thing I like is the exercises at the end of each chapter. These exercises are a great way to reinforce the main points of each chapter and force you to get your hands dirty.”
- Hien Luu, SD Forum/Java SIG
“I loved the way this book is set up. We used this in my Java Programming class, and it just makes everything so much easier and clearer.”
- Posted at an online bookseller
“I've most recently used the Java book to supplement my (rather terrible) textbook for a recent Java class at a local college. I made an A in the class, which wouldn't have been possible with the required textbook alone.”
- IT Manager, North Carolina
“The beauty of Murach books is that they are broken into what I call 'mini lessons.’ I can look at a chapter and decide it has 14 mini lessons, and that is manageable to me as opposed to saying I need to read 30 pages to cover the chapter. In addition, each mini lesson is 2 pages. The left page describes the material and the right page provides the examples. No longer do I have to hunt for the examples in the discussion. And the book is less than $100. This was a true bargain in the runaway prices of textbooks in colleges these days, especially in the STEM subjects.”
- Posted at an online bookseller
“I have worked for several training companies as a trainer, and I can categorically state that Murach Books are superior to any courseware that I have ever encountered. Your examples and code are more hands-on, and they work. You may quote me.”
- Robert Vaughn, PhD, Iowa
View the table of contents for this book in a PDF: Table of Contents (PDF)
Click on any chapter title to display or hide its content.
Java timeline
Java editions
How Java compares to C++ and C#
Two types of desktop applications
Web applications and mobile apps
The code for a console application
How Java compiles and interprets code
How to use the command prompt to compile and run a Java application
An introduction to Java IDEs
An introduction to NetBeans
How to open and close a project
How to compile and run a project
How to work with the Output window
How to create a new project
An introduction to Eclipse
How to open and close a project
How to compile and run a project
How to work with the Console window
How to create a new project
How to declare a class and a main() method
How to code statements
How to code comments
How to declare and initialize variables
Rules and recommendations for naming variables
How to code arithmetic expressions
How to declare and initialize a string
How to join and append strings
How to include special characters in strings
How to import classes
How to create objects and call methods
How to print output to the console
How to read input from the console
How to compare numeric variables
How to compare string variables
How to code if/else statements
How to code while statements
The Invoice application
The Test Score application
How to test and debug an application
How to view the documentation for a class
The eight primitive data types
How to declare and initialize variables
How to declare and initialize constants
How to use the binary operators
How to use the compound assignment operators
How to use the unary operators
How to work with the order of precedence
How to work with casting
How to use the Math class
How to use the NumberFormat class
How to print formatted output to the console
The Invoice application with formatted output
How to debug a rounding error
How to use the relational operators
How to use the logical operators
How to code if/else statements
How to work with braces
How to code nested if/else statements
How to code switch statements
How to use arrow labels with switch statements
How to code switch expressions
The Invoice application with a switch expression
How to code while loops
How to code do-while loops
How to code for loops
The Future Value application
How to code nested loops
How to code break statements
How to code continue statements
The Guess the Number application
How to code static methods
How to call static methods
The Future Value application with a static method
The Guess the Number application with static methods
How exceptions work
How to catch exceptions
How to prevent exceptions
How to validate a single entry
How to code a method that validates an entry
The console
The code
Typical test phases
The three types of errors
Common Java errors
A simple way to trace code execution
How to set and remove breakpoints
How to step through code
How to inspect variables
How to inspect the stack trace
How to set and remove breakpoints
How to step through code
How to inspect variables
How to inspect the stack trace
How classes can be used to structure an application
How encapsulation works
The relationship between a class and its objects
The Product class
How to code instance variables
How to code constructors
How to code methods
How to create an object and call its methods
How to code static fields and methods
How to call static fields and methods
The ProductDB class
The console
The code
Reference types compared to primitive types
How to code a copy constructor
How to overload methods
How to use the this keyword
The console
The class diagram
The code
How to create an array
How to assign values to an array
How to use loops with arrays
How to work with rectangular arrays
How to fill, sort, and search arrays
How to refer to, copy, and compare arrays
The Number Cruncher application
A comparison of arrays and array lists
How to create an array list
How to add and get elements
How to replace, remove, and search for elements
How to store primitive values in an array list
The console
The Invoice class
The InvoiceApp class
How files and streams work
A file I/O example
How to use a try-with-resources statement to handle I/O exceptions
How to connect a character output stream to a file
How to write to a text file
How to connect a character input stream to a file
How to read from a text file
The ProductDB class
The console
The ProductManagerApp class
The exception hierarchy
How exceptions are propagated
How to declare a method that throws an exception
How to throw an exception
How to use the methods of an exception
How to code a finally block
How inheritance works
How the Object class works
How to create a superclass
How to create a subclass
How polymorphism works
The console
The Product class
The Book and Software classes
The ProductDB class
The ProductApp class
How to cast objects
How to check the type of an object
How to compare objects for equality
How to define a custom exception
How to work with abstract classes
How to work with the final classes, methods, and parameters
How to work with sealed classes
An introduction to records
How to use and customize records
When to use inheritance
When to use composition
A simple interface
Interfaces compared to abstract classes
How to code an interface
How to implement an interface
How to inherit a class and implement an interface
How to use an interface as a parameter
How to work with default and static methods
How to declare an enumeration
How to use an enumeration
How to enhance an enumeration
An introduction to packages
How to use NetBeans to work with packages
How to use Eclipse to work with packages
How to work with libraries
An introduction to the module system
How to create and use modules
How to add javadoc comments to a class
How to generate Java documentation
How to view the documentation
A GUI that displays ten controls
A summary of GUI frameworks
The inheritance hierarchy for JavaFX nodes
How to use NetBeans
How to use Eclipse
How to create and display a window
How to work with labels
How to set alignment and padding
How to work with text fields
How to set column widths
How to work with buttons and boxes
How to handle action events
The Future Value application
How to display an error message in a dialog box
How to validate the data entered into a text field
The Validation class
How to validate multiple entries
How to work with check boxes
How to work with radio buttons
How to work with combo boxes
How to work with date pickers
How to work with list views
How to work with text areas and scroll bars
The user interface
The code
How to compare strings
How to work with string indexes
How to modify strings
How to work with the characters in a string
The user interface
The CreateAccountApp class
How to work with text blocks
How to split and join strings
How to format strings
The user interface
The WordList class
The Hangman class
The HangmanApp class
An introduction to StringBuilder objects
How to use a StringBuilder object with a loop
The Java collection framework
More about generics
How to create a linked list and add and get elements
How to replace, remove, and search for elements
The Invoice application
How to sort a collection of numbers or strings
How to sort objects by implementing the Comparable interface
How to sort objects with a custom comparator
How to work with a stack
How to work with a queue
How to use generics to create a custom collection
The HashMap and TreeMap classes
Code examples that work with maps
The Word Counter application
Anonymous classes compared to lambdas
Pros and cons of lambda expressions
A method that doesn’t use lambdas
A method that uses lambdas
The syntax of a lambda expression
How to use the Predicate interface
How to use the Consumer interface
How to use the Function interface
How to work with multiple functional interfaces
How to filter a list
How to map a list
How to reduce a list
How to add a range of numbers
How to compute the factorial of a number
How to implement the binary search algorithm
How to calculate a value in the Fibonacci series
How to decide if you should use recursion
How to use the File class
The algorithm
A directory tree
The console
The code
The date/time API prior to Java 8
The date/time API for Java 8 and later
How to create date and time objects
How to get date and time parts
How to compare dates and times
How to adjust dates and times
How to add or subtract a period of time
How to get the time between two dates
How to format dates and times
An Invoice class that includes an invoice date
How a table is organized
How the columns in a table are defined
How tables are related
How to query a single table
How to join data from two or more tables
How to add, update, and delete data in a table
An introduction to SQLite
How to use DB Browser to view and edit a table in a SQLite database
How to use DB Browser to run SQL statements
How to create a SQLite database
Four types of JDBC database drivers
How to download a database driver
How to add a database driver to a project
How to connect to a database
How to return a result set and move the cursor through it
How to get data from a result set
How to work with prepared statements
How to insert, update, and delete data
The ProductDB class
Code that uses the ProductDB class
How to install the JDK
How to install the source code for this book
How to install Netbeans
How to install Eclipse
How to install DB Browser for SQLite
How to install the JDK
How to install the source code for this book
How to install Netbeans
How to install Eclipse
How to install DB Browser for SQLite
Unlike other publishers, we don’t fill dozens of pages in our books with end-of-chapter activities that may never be used. Instead, we provide everything you need for an effective course in a download from our instructor’s website. Then, you decide which of these materials you want to use. In short, our instructor’s materials provide a turnkey package for a powerful Java course.
For a detailed description of all the materials, please see the Instructor’s Summary PDF. But here’s a quick overview:
On this page, we’ll be posting answers to the questions that come up most often about this book. So if you have any questions that you haven’t found answered here at our site, please email us. Thanks!
There are no book corrections that we know of at this time. But if you find any, please email us, and we’ll post any corrections that affect the technical accuracy of the book here. Thank you!
This is our site for college instructors. To buy Murach books, please visit our retail site.